Overview
In this lab, we will present a scenario where Visual Studio Team Services (VSTS) can enable Node.js developers with continuous integration and deployment features. The scenario demonstrates how a Node.js developer using Visual Studio Code and various Azure-related VS Code extensions to create a new Azure App Service and use Git-based deployment. You’ll learn how a Node.js developer can use VSTS to ensure that their code is deployed automatically to an Azure App Service when code is committed to a VSTS Git repository.
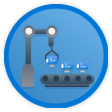
Prerequisites
- If you don’t already have a Visual Studio Dev Essentials and Visual Studio Team Services account, create one at my.visualstudio.com.
- If you don’t already have an Azure subscription, create one here.
- Install Git if you don’t already have it installed.
- Install Node.js either by using the downloadable installers from Node.js or using various command-line tools. Mac users can install Node.js using Homebrew using the command
brew install node
. - Install Visual Studio Code.
- Install the Azure App Service Tools using the VS Code Extensions Palette (search for azure).
-
Once you have installed Visual Studio Code and the App Service Tools extension, open Visual Studio Code. In Code, use
Ctrl-Shift-P
(orCmd-Shift-P
on a Mac) to open up the command palette. Type the wordpath
to filter the command menu, and then select the command Install ‘code’ command in PATH to make it easy to open Visual Studio Code from your command line.
Demo Steps
Express is a minimal, flexible web application framework for Node.js developers that provides a robust set of features for both web and mobile web developers. The Express package is available via NPM, easily installable into any Node.js application using the command npm install express
. In most cases Express needn’t be installed as a global package using the -g
NPM switch, as the convention is that Express is installed independently into each Node.js app during development.
This demo will walk you through the process of creating a new Node.js web app that uses Express. Then you’ll create a new Visual Studio Team Services project to use for storing the code and continuously deploying the app to Azure App Service.
Scaffolding a new Express app using Yeoman
To ease the process of bootstrapping a Node.js Express application, developers can use the Yeoman template engine. Yeoman - known as yo from it’s NPM CLI command name - has hundreds of scaffolders for all sorts of projects. Luckilly, Yeoman is easilly installable as an NPM package just like Express and many other Node.js components.
Install Yeoman and the Express generator
-
Run the command
npm install -g yo
. -
Type
yo
at the command prompt. -
Type
express
at the prompt and hit enter to search the Yeoman template database for Express-related scaffolders. -
Scroll through the list of results until you see the generator named, simply, express, and then hit enter.
Generate a skeleton Express app
-
In the command prompt, enter the command
yo express
to create a new Express application. -
Enter the command
code .
to start Visual Studio Code with the new Express app’s folder open. -
Edit
gulpfile.js
to turn off local hosting during the Gulp build. The code for thedefault
task should be commented out:gulp.task('default', [ //'develop' ]);
Create an Azure App Service using VS Code
There are a number of various extensions for VS Code that provide Azure functionality. Extensions like the Azure CLI Tools make it easy to write scripts that make use of the Azure CLI to perform administrative tasks in your Azure nn.
The Azure App Service Tools for VS Code provide a series of convenient features for creating new Azure App Services.
Login to your Azure subscription
-
Expand the Azure App Service Tools pane. Click the
Sign in to Azure
gesture in the explorer pane. -
Click
Copy and Open
to open the browser and log in to your Azure subscription. -
Hit
Ctrl-V
orCmd-V
on Mac, or use the context menu to paste the login code into the textbox. -
Click the Continue button to perform the login routine.
Once you click Continue, you’ll be able to select which of the organizational or Microsoft accounts you want to use to login to your Azure subscription.
-
Once the login page loads, flip back to VS Code. You should see all of your Azure subscriptions in the App Service pane. When you expand the pane, all of your App Services are visible.
Create the App Service Web App
To setup continuous deployment to an Azure App Service, an Azure App Service resource must be created into which code can be deployed. Luckilly, Visual Studio, Visual Studio for Mac, and Visual Studio Code all make this a rather easy task directly within the various IDEs.
-
In VS Code, right-click the subscription you want to use and select the
Create New Web App
menu option. -
Type in a name for the App Service. Note, if an existing App Service with this name exists you’ll be informed with an error message.
-
Create a new resource group for the App Service.
-
Give the new resource group a name.
-
Select the geographic region from the menu.
-
Select the option for creating a new App Service Plan.
-
Give the new App Service Plan a name.
-
Select the tier of service.
-
Select the Node.js version your app will need. VS Code will try to determine this for you but you have the option to choose your version.
-
Visual Studio Code will provide a stream of information as the resources are being created in your subscription.
-
Once the App Service is created, it will appear in the App Service Explorer pane
Viewing the new App Service in the Azure Portal
-
In VS Code, right-click on the App Service to open the context menu. Select the
Open in Portal
option to open the new App Service in the Azure Portal. -
Once you select an App Service to view within VS Code it’ll open directly in the Azure portal in your default browser. Click the link in the portal’s overview section to open the new App Service in a separate tab.
-
The App Service should open in your browser, displaying the default App Service start page.
Use Team Services to Enable Continuous Deployment
Now that the app is ready to be deployed we’ll set up a new Team Services project and set it up with Git so the code can be easily committed and continuously deployed.
Create the new Team Services Project
-
Browse to your Team Services account at https://{youraccount}.visualstudio.com. Give the project a name and click Create.
-
If you haven’t already created Git credentials click the Generate Git credentials button, then provide a username and password to be used when interacting with this project. Then, click the Copy clone url to clipboard button as shown below.
-
At a command prompt in your project’s folder, type the command
git init
to initialize a new repository locally. Then, typegit remote add origin {copied URL}
to add the remote to your local repository. Then,add
andcommit
the files to the repository. Finally, usegit push origin master
to push the Express app code up to the VSTS project’s Git repository. -
Click on the Code tab in your Team Services browser.
Create the Build Definition
-
Click the Set up build button in the Files page in the browser.
-
Find the build template named NodeJS with Gulp and click the Apply button.
-
Give the build definition the name
DevDeploy
and select Hosted from the Default agent queue menu. -
Click the Copy Publish Artifact: drop build task to see the details of this task.
-
Click the Add Task button under the list of build tasks. Select the Azure App Service Deploy task and click the Add button next to it.
-
Note that the Azure subscription and App Service name menus are both required.
-
Select the Azure subscription in which your destination App Service is housed.
-
Select the App Service you created earlier within VS Code to use as the deployment target for your Node.js source code.
-
Click the previous build task (the “Copy Publish Artifact: drop” task) and take note of the Copy root and Contents text boxes.
-
Once you’ve copied these two value and pasted them both into the Deploy build task, the value of the task’s Package or folder textbox should represent the full path the ZIP file being built by your VSTS build.
-
Check the checkbox labelled Generate Web.config to enable that section of the task window. Then click the ellipse button next to the text box to open up the Web.config parameters dialog.
-
Select node from the Web.config parameters screen’s Application framework menu. Then, change the NodeStartFile value to be
app.js
since the Express app’s default filename isapp.js
.
Run the Build
Now the build definition is complete running it will result in the code being deployed to the Azure App Service.
-
To kick off the manual build, click the Save and Queue spin button in the build definition page. Then, select the Save and queue option to save the definition and trigger the build.
-
Click the Queue button on the Queue build for deploy dialog.
-
Click the queued build link to drill into the running build.
-
The build log is visible in the browser.
-
Wait until the build has completed.
-
Open up a browser (or refresh the already-open browser tab) on the App Service URL.
Enabling triggered builds
-
Click the Triggers tab in Team Services.
-
Edit the code in
controllers/home.js
so that thearticles
variable in theget
method is instantiated using the code below.var articles = [ new Article({ 'title': 'First Article', 'text' : 'This is the text of the first article' }), new Article({ 'title': 'Second Article', 'text' : 'This is the text of the second article' }) ];
-
Edit the code in
view\index.handlebars
to add adl
list containing a presentation of the articles in the view model. -
Commit the code to the repository.
-
Go back to the build definition in Team Services and take note that a new build has been queued.
-
Refresh the site in the browser.